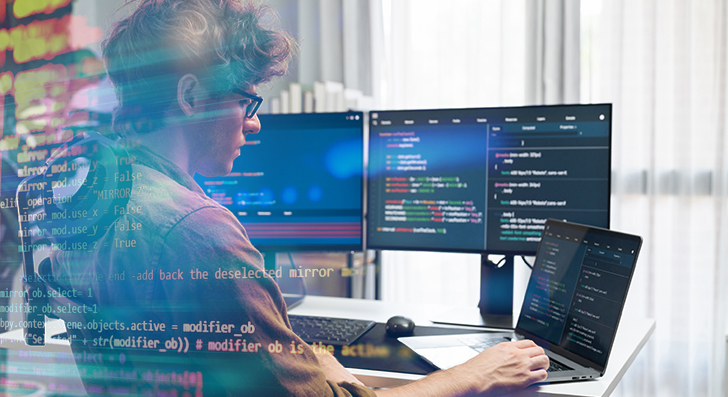
Scalability usually means your application can handle expansion—a lot more customers, extra facts, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in your mind saves time and worry later on. Right here’s a clear and sensible guideline that may help you start by Gustavo Woltmann.
Style and design for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be component within your prepare from the beginning. Many apps fail when they develop speedy mainly because the original design can’t cope with the additional load. As being a developer, you might want to Consider early regarding how your method will behave stressed.
Start by planning your architecture to be adaptable. Steer clear of monolithic codebases wherever every thing is tightly related. Instead, use modular layout or microservices. These styles break your app into more compact, independent areas. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from working day a person. Will it need to deal with 1,000,000 end users or simply just 100? Choose the correct style—relational or NoSQL—based on how your information will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional crucial position is to stay away from hardcoding assumptions. Don’t write code that only functions below recent ailments. Give thought to what would materialize In the event your person foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or celebration-pushed programs. These support your app cope with additional requests devoid of finding overloaded.
Any time you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be lessening upcoming complications. A properly-planned method is less complicated to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the Right Databases
Picking out the appropriate database is a vital Component of creating scalable applications. Not all databases are crafted the same, and utilizing the Improper one can sluggish you down or perhaps cause failures as your application grows.
Begin by understanding your facts. Is it extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is a good in good shape. These are generally powerful with interactions, transactions, and consistency. In addition they guidance scaling methods like browse replicas, indexing, and partitioning to deal with more targeted traffic and information.
If the information is a lot more flexible—like consumer activity logs, product or service catalogs, or documents—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured information and might scale horizontally more simply.
Also, contemplate your browse and create designs. Are you presently carrying out numerous reads with fewer writes? Use caching and read replicas. Do you think you're managing a hefty generate load? Consider databases that could tackle higher publish throughput, or simply event-based mostly facts storage units like Apache Kafka (for temporary info streams).
It’s also good to think ahead. You may not need to have State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change afterwards.
Use indexing to hurry up queries. Avoid needless joins. Normalize or denormalize your knowledge based upon your access patterns. And usually check databases effectiveness as you develop.
In brief, the correct database is determined by your app’s structure, speed needs, and how you expect it to grow. Get time to pick wisely—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every small hold off provides up. Improperly penned code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s crucial to build efficient logic from the beginning.
Start off by composing thoroughly clean, basic code. Stay away from repeating logic and remove anything avoidable. Don’t pick the most intricate Resolution if a simple 1 is effective. Maintain your functions shorter, targeted, and easy to check. Use profiling equipment to find bottlenecks—sites the place your code requires much too extended to operate or works by using a lot of memory.
Subsequent, evaluate your database queries. These often sluggish issues down much more than the code by itself. Be certain Every single question only asks for the information you truly require. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across massive tables.
If you recognize the same knowledge being requested time and again, use caching. Store the outcome briefly applying resources like Redis or Memcached and that means you don’t really have to repeat costly operations.
Also, batch your databases operations once you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and makes your app far more economical.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred information may well crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when needed. These actions aid your application continue to be smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more customers and much more site visitors. If every little thing goes by means of a single server, it is going to speedily turn into a bottleneck. That’s wherever load balancing and caching are available. Both of these instruments support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out every one of the operate, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to create.
Caching is about storing information quickly so it could be reused rapidly. When users ask for the identical information yet again—like a product web site or maybe a profile—you don’t must fetch it from the databases each time. You are able to provide it from your cache.
There's two frequent types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near to the person.
Caching lowers databases load, enhances velocity, and tends to make your application much more successful.
Use caching for things that don’t modify normally. And constantly be certain your cache is up to date when details does alter.
In short, load balancing and caching are basic but impressive resources. Jointly, they help your app take care of more consumers, keep fast, and Recuperate from challenges. If you plan to expand, you require both.
Use Cloud and Container Instruments
To build scalable applications, you may need instruments that permit your app develop simply. That’s wherever cloud platforms and containers are available. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential capability. When targeted traffic boosts, you may increase extra means with just some clicks or quickly using vehicle-scaling. When visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You'll be able to concentrate on developing your app instead of running infrastructure.
Containers are A different critical Device. A container deals your app and all the things it really should operate—code, libraries, configurations—into one particular unit. This can make it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes assist you manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, and that is great for effectiveness and reliability.
To put it briefly, making use of cloud and container resources usually means you'll be able to scale fast, deploy simply, and recover speedily when problems come about. If you want your app to mature without having restrictions, begin working with these tools early. They preserve time, cut down threat, and make it easier to stay focused on building, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go Incorrect. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better choices as your app grows. It’s a critical Element of developing scalable techniques.
Get started by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this facts.
Don’t just observe your servers—monitor your app as well. Keep watch over how long it will take for consumers to load web pages, how often problems come about, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital complications. Such as, In the event your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you must get notified right away. This aids you repair problems fast, normally right before people even observe.
Monitoring can be beneficial whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it read more back again prior to it results in authentic injury.
As your app grows, website traffic and knowledge improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the correct tools in position, you remain in control.
Briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not pretty much spotting failures—it’s about understanding your technique and making sure it really works well, even under pressure.
Final Ideas
Scalability isn’t only for huge providers. Even tiny applications require a robust foundation. By planning carefully, optimizing correctly, and utilizing the correct tools, it is possible to build apps that improve smoothly with no breaking stressed. Begin modest, think huge, and Make smart.